In my work as an Android developer, I frequently get across situations when I need to call a function from one activity to another. Understanding how to complete this activity effectively is crucial, whether it involves altering UI components or initiating particular activities. I'll walk you through a quick, step-by-step way in this tutorial for utilizing code snippets to call a function in Android Studio from another activity.
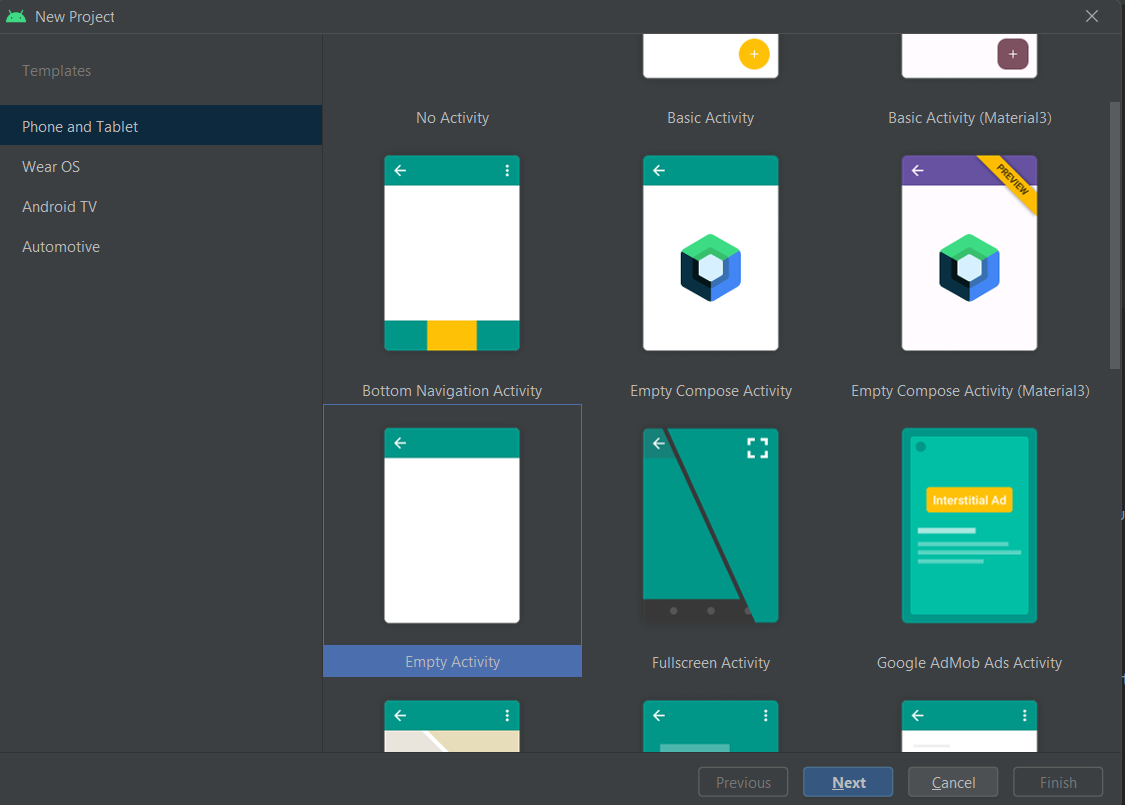
Step 1: Define the Method in the Source Activity First, let's define the method we want to call in the source activity. This method should perform the desired action or task that we want to execute from another activity. For example, let's say we have a method named "updateUI()" in our source activity.
public class SourceActivity extends AppCompatActivity {
// Define the method to be called
public void updateUI() {
// Perform the desired action here
}
}
Step 2: Navigate to the Destination Activity Next, we need to navigate to the destination activity from where we want to call the method defined in the source activity. This can be achieved by creating an Intent and starting the destination activity.
Intent intent = new Intent(SourceActivity.this, DestinationActivity.class);
startActivity(intent);
Step 3: Call the Method from the Destination Activity Now that we're in the destination activity, we can call the method defined in the source activity. To do this, we'll need to obtain an instance of the source activity and then call the method using that instance.
SourceActivity sourceActivity = new SourceActivity();
sourceActivity.updateUI();
Step 4: Handle Exceptions It's important to handle potential exceptions that may arise when calling the method from another activity. For example, if the source activity is not currently active or has been destroyed, attempting to call the method may result in a NullPointerException. To prevent this, we can add appropriate error handling mechanisms.
try {
SourceActivity sourceActivity = new SourceActivity();
sourceActivity.updateUI();
} catch (NullPointerException e) {
e.printStackTrace();
// Handle the exception gracefully
}
Conclusion: By following these simple steps, you can easily call a method from another activity in Android Studio. Whether you're updating UI elements, triggering actions, or performing any other task, this technique allows for seamless communication between different activities in your Android application. With practice and experimentation, you'll become adept at integrating this functionality into your projects and enhancing the user experience.